This tutorils will teach about complete library management project.the purpose of this project is to develop a rest api using Spring boot to manage author,publisher,books,user,borrowel details.
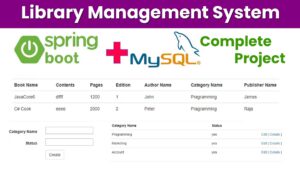
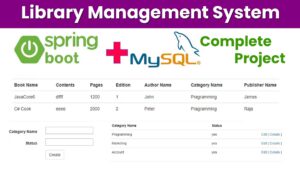
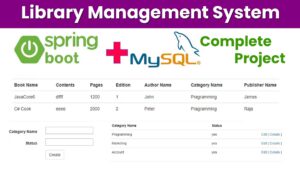
Lets Started Project
Project Set up
First Step go to Spring Initializer to configure the project using the following dependencies.
1. Spring Data JPA
2. Spring Web
3. Lombok
4. MySQL Driver
Create the Package entity
Inside the Package create the class Author
Author
@Entity @Table(name="author") public class Author { @Id @GeneratedValue(strategy = GenerationType.AUTO) @Column(name = "author_id",length = 11) private int authorid; @Column(name = "name",length = 45) private String name; @OneToMany(mappedBy = "author") private Set<Book> books; public Author(int authorid, String name) { this.authorid = authorid; this.name = name; } public Author(String name) { this.name = name; } public Author() { } public int getAuthorid() { return authorid; } public void setAuthorid(int authorid) { this.authorid = authorid; } public String getName() { return name; } public void setName(String name) { this.name = name; } @Override public String toString() { return "Author{" + "authorid=" + authorid + ", name='" + name + '\'' + '}'; } }
Create the Package DTO
Inside the Package create the class AuthorDTO and AuthorSaveDTO and AuthorUpdateDTO
AuthorDTO
@AllArgsConstructor @NoArgsConstructor @Data public class AuthorDTO { private int authorid; private String name; }
AuthorSaveDTO
@AllArgsConstructor @NoArgsConstructor @Data public class AuthorSaveDTO { private String name; }
AuthorUpdateDTO
@AllArgsConstructor @NoArgsConstructor @Data public class AuthorUpdateDTO { private int authorid; private String name; }
Create the Package Controller
inside the Package create the class AuthorController
@RestController @CrossOrigin @RequestMapping("api/v1/author") public class AuthorController { @Autowired private AuthorService authorService; @PostMapping(path = "/save") public String saveAuthor(@RequestBody AuthorSaveDTO authorSaveDTO) { String authorname = authorService.addAuthor(authorSaveDTO); return "Added Successfully"; } @GetMapping(path = "/getAllAuthor") public List<AuthorDTO> getAllAuthor() { List<AuthorDTO> allAuthors = authorService.getAllAuthor(); return allAuthors; } @PutMapping(path = "/update") public String updateAuthor(@RequestBody AuthorUpdateDTO authorUpdateDTO) { String authorname = authorService.updateAuthor(authorUpdateDTO); return authorname; } @DeleteMapping(path = "/delete/{id}") public String deleteAuthor(@PathVariable(value = "id")int id) { String authorname = authorService.deleteAuthor(id); return "deleteddd"; } }
Create the Package Author Service
Inside the Package create the interface AuthorService.java and AuthorServiceIMPL.java.
AuthorService
public interface AuthorService { String addAuthor(AuthorSaveDTO authorSaveDTO); List<AuthorDTO> getAllAuthor(); String updateAuthor(AuthorUpdateDTO authorUpdateDTO); String deleteAuthor(int id); }
AuthorServiceIMPL
@Service public class AuthorServiceIMPL implements AuthorService { @Autowired private AuthorRepo authorRepo; @Override public String addAuthor(AuthorSaveDTO authorSaveDTO) { Author author = new Author( authorSaveDTO.getName() ); authorRepo.save(author); return author.getName(); } @Override public List<AuthorDTO> getAllAuthor() { List<Author> getAuthors = authorRepo.findAll(); List<AuthorDTO> authorDTOList = new ArrayList<>(); for(Author author : getAuthors) { AuthorDTO authorDTO = new AuthorDTO( author.getAuthorid(), author.getName() ); authorDTOList.add(authorDTO); } return authorDTOList; } @Override public String updateAuthor(AuthorUpdateDTO authorUpdateDTO) { if (authorRepo.existsById(authorUpdateDTO.getAuthorid())) { Author author = authorRepo.getById(authorUpdateDTO.getAuthorid()); author.setName(authorUpdateDTO.getName()); authorRepo.save(author); return author.getName(); } else { System.out.println("Author ID Not Exist!!!!!!!!"); } return null; } @Override public String deleteAuthor(int id) { if(authorRepo.existsById(id)) { authorRepo.deleteById(id); } else { System.out.println("ID Not Found"); } return null; } }
Create the Package Repo
AuthorRepo
public interface AuthorRepo extends JpaRepository<Author,Integer > { }
Entity
Inside the Package create the class Publisher
Publisher
@Entity @Table(name="publisher") public class Publisher { @Id @GeneratedValue(strategy = GenerationType.AUTO) @Column(name = "publisher_id",length = 11) private int publisherid; @Column(name = "name",length = 45) private String name; @OneToMany(mappedBy = "publisher") private Set<Book> books; public Publisher(int publisherid, String name) { this.publisherid = publisherid; this.name = name; } public Publisher(String name) { this.name = name; } public Publisher() { } public int getPublisherid() { return publisherid; } public void setPublisherid(int publisherid) { this.publisherid = publisherid; } public String getName() { return name; } public void setName(String name) { this.name = name; } @Override public String toString() { return "Publisher{" + "publisherid=" + publisherid + ", name='" + name + '\'' + '}'; } }
PublisherDTO
@AllArgsConstructor @NoArgsConstructor @Data public class PublisherDTO { private int publisherid; private String name; }
PublisherSaveDTO
@AllArgsConstructor @NoArgsConstructor @Data public class PublisherSaveDTO { private String name; }
PublisherUpdateDTO
@AllArgsConstructor @NoArgsConstructor @Data public class PublisherUpdateDTO { private int publisherid; private String name; }
Create the Package Controller
inside the Package create the class PublisherController
PublisherController
@RestController @CrossOrigin @RequestMapping("api/v1/publisher") public class PublisherController { @Autowired private PublisherService publisherService; @PostMapping(path = "/save") public String savePublisher(@RequestBody PublisherSaveDTO publisherSaveDTO) { String publishername = publisherService.addPublisher(publisherSaveDTO); return "Added Successfully"; } @GetMapping(path = "/getAllPublisher") public List<PublisherDTO> getAllAuthor() { List<PublisherDTO> allPublishers = publisherService.getAllPublisher(); return allPublishers; } @PutMapping(path = "/update") public String updatePublisher(@RequestBody PublisherUpdateDTO publisherUpdateDTO) { String publishername = publisherService.updatePublisher(publisherUpdateDTO); return publishername; } @DeleteMapping(path = "/delete/{id}") public String deletePublisher(@PathVariable(value = "id")int id) { String authorname = publisherService.deletePublisher(id); return "deleteddd"; } }
Create the Package Publisher Service
Inside the Package create the interface PublisherService.java and PublisherServiceIMPL.java.
PublisherService
public interface PublisherService { String addPublisher(PublisherSaveDTO publisherSaveDTO); List<PublisherDTO> getAllPublisher(); String updatePublisher(PublisherUpdateDTO publisherUpdateDTO); String deletePublisher(int id); }
PublisherServiceIMPL
@Service public class PublisherServiceIMPL implements PublisherService { @Autowired private PublisherRepo publisherRepo; @Override public String addPublisher(PublisherSaveDTO publisherSaveDTO) { Publisher publisher = new Publisher( publisherSaveDTO.getName() ); publisherRepo.save(publisher); return publisher.getName(); } @Override public List<PublisherDTO> getAllPublisher() { List<Publisher> getPublishers = publisherRepo.findAll(); List<PublisherDTO> publisherDTOList = new ArrayList<>(); for(Publisher publisher : getPublishers) { PublisherDTO publisherDTO = new PublisherDTO( publisher.getPublisherid(), publisher.getName() ); publisherDTOList.add(publisherDTO); } return publisherDTOList; } @Override public String updatePublisher(PublisherUpdateDTO publisherUpdateDTO) { if (publisherRepo.existsById(publisherUpdateDTO.getPublisherid())) { Publisher publisher = publisherRepo.getById(publisherUpdateDTO.getPublisherid()); publisher.setName(publisherUpdateDTO.getName()); publisherRepo.save(publisher); return publisher.getName(); } else { System.out.println("Publisher ID Not Exist!!!!!!!!"); } return null; } @Override public String deletePublisher(int id) { if(publisherRepo.existsById(id)) { publisherRepo.deleteById(id); } else { System.out.println("ID Not Found"); } return null; } }
PublisherRepo
public interface PublisherRepo extends JpaRepository<Publisher,Integer > { }
entity
Inside the Package create the class Book
@Entity @Table(name="book") public class Book { @Id @GeneratedValue(strategy = GenerationType.AUTO) @Column(name = "book_id",length = 11) private int bookid; @Column(name = "book_title",length = 45) private String title; @ManyToOne @JoinColumn(name = "author_id") private Author author; @ManyToOne @JoinColumn(name = "publisher_id") private Publisher publisher; @OneToMany(mappedBy = "book") private Set<Borrow> borrows; public Book(int bookid, String title, Author author, Publisher publisher) { this.bookid = bookid; this.title = title; this.author = author; this.publisher = publisher; } public Book(String title, Author author, Publisher publisher) { this.title = title; this.author = author; this.publisher = publisher; } public Book() { } public int getBookid() { return bookid; } public void setBookid(int bookid) { this.bookid = bookid; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public Author getAuthor() { return author; } public void setAuthor(Author author) { this.author = author; } public Publisher getPublisher() { return publisher; } public void setPublisher(Publisher publisher) { this.publisher = publisher; } @Override public String toString() { return "Book{" + "bookid=" + bookid + ", title='" + title + '\'' + ", author=" + author + ", publisher=" + publisher + '}'; } }
BookDTO
Inside the Package create the class BookDTO and BookSaveDTO and BookUpdateDTO
BookDTO
@AllArgsConstructor @NoArgsConstructor @Data public class BookDTO { private int bookid; private String title; private Author author; private Publisher publisher; }
BookSaveDTO
@AllArgsConstructor @NoArgsConstructor @Data public class BookSaveDTO { private String title; private int author_id; private int publisher_id; }
BookUpdateDTO
@AllArgsConstructor @NoArgsConstructor @Data public class BookUpdateDTO { private int bookid; private String title; private int author_id; private int publisher_id; }
Controller
Inside the Package create the class BookController
@RestController @CrossOrigin @RequestMapping("api/v1/book") public class BookController { @Autowired private BookService bookService; @PostMapping(path = "/save") public String saveBook(@RequestBody BookSaveDTO bookSaveDTO) { String booktitle = bookService.addBook(bookSaveDTO); return "Added Successfully"; } @GetMapping(path = "/getAllBook") public List<BookDTO> getAllBook() { List<BookDTO> allBooks = bookService.getAllBook(); return allBooks; } @PutMapping(path = "/update") public String updateBook(@RequestBody BookUpdateDTO bookUpdateDTO) { String bookname = bookService.updateBook(bookUpdateDTO); return bookname; } @DeleteMapping(path = "/delete/{id}") public String deleteBook(@PathVariable(value = "id")int id) { String Bookname = bookService.deleteBook(id); return "deleteddd"; } }
Inside the Package create the interface BookService.java and BookServiceIMPL.java.
BookService
public interface BookService { String addBook(BookSaveDTO bookSaveDTO); List<BookDTO> getAllBook(); String updateBook(BookUpdateDTO bookUpdateDTO); String deleteBook(int id); }
BookServiceIMPL
@Service public class BookServiceIMPL implements BookService { @Autowired private AuthorRepo authorRepo; @Autowired private PublisherRepo publisherRepo; @Autowired private BookRepo bookRepo; @Override public String addBook(BookSaveDTO bookSaveDTO) { Book book = new Book( bookSaveDTO.getTitle(), authorRepo.getById(bookSaveDTO.getAuthor_id()), publisherRepo.getById(bookSaveDTO.getPublisher_id()) ); bookRepo.save(book); return book.getTitle(); } @Override public List<BookDTO> getAllBook() { List<Book> getBooks = bookRepo.findAll(); List<BookDTO> bookDTOList = new ArrayList<>(); for(Book book : getBooks) { BookDTO bookDTO = new BookDTO( book.getBookid(), book.getTitle(), book.getAuthor(), book.getPublisher() ); bookDTOList.add(bookDTO); } return bookDTOList; } @Override public String updateBook(BookUpdateDTO bookUpdateDTO) { if(bookRepo.existsById(bookUpdateDTO.getBookid())) { Book book = bookRepo.getById(bookUpdateDTO.getBookid()); book.setTitle(bookUpdateDTO.getTitle()); book.setAuthor(authorRepo.getById(bookUpdateDTO.getAuthor_id())); book.setPublisher(publisherRepo.getById(bookUpdateDTO.getPublisher_id())); bookRepo.save(book); return book.getTitle(); } else { System.out.println("Book ID Not Found"); } return null; } @Override public String deleteBook(int id) { if(bookRepo.existsById(id)) { bookRepo.deleteById(id); } else { System.out.println("Book ID Not Found"); } return null; } }
BookRepo
public interface BookRepo extends JpaRepository<Book,Integer > { }
Borrow
Inside the Package create the class borrow
@Entity @Table(name="borrow") public class Borrow { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private int id; @ManyToOne @JoinColumn(name = "book_id") private Book book; @ManyToOne @JoinColumn(name = "user_id") private User user; @Column(name = "borrowDate") private LocalDate borrowDate; @Column(name = "returnDate") private LocalDate returnDate; @OneToMany(mappedBy = "borrow") private Set<Fine> fines; public Borrow(int id, Book book, User user, LocalDate borrowDate, LocalDate returnDate) { this.id = id; this.book = book; this.user = user; this.borrowDate = borrowDate; this.returnDate = returnDate; } public Borrow(Book book, User user, LocalDate borrowDate, LocalDate returnDate) { this.book = book; this.user = user; this.borrowDate = borrowDate; this.returnDate = returnDate; } public Borrow() { } public int getId() { return id; } public void setId(int id) { this.id = id; } public Book getBook() { return book; } public void setBook(Book book) { this.book = book; } public User getUser() { return user; } public void setUser(User user) { this.user = user; } public LocalDate getBorrowDate() { return borrowDate; } public void setBorrowDate(LocalDate borrowDate) { this.borrowDate = borrowDate; } public LocalDate getReturnDate() { return returnDate; } public void setReturnDate(LocalDate returnDate) { this.returnDate = returnDate; } @Override public String toString() { return "Borrow{" + "id=" + id + ", book=" + book + ", user=" + user + ", borrowDate=" + borrowDate + ", returnDate=" + returnDate + '}'; } }
BorrowDTO
Inside the Package create the class borrowDTO and borrowSaveDTO and borrowUpdateDTO
borrowDTO
@AllArgsConstructor @NoArgsConstructor @Data public class BorrowDTO { private int id; private Book book; private User user; private LocalDate borrowDate; private LocalDate returnDate; }
borrowSaveDTO
@AllArgsConstructor @NoArgsConstructor @Data public class BookSaveDTO { private String title; private int author_id; private int publisher_id; }
borrowUpdateDTO
@AllArgsConstructor @NoArgsConstructor @Data public class BookUpdateDTO { private int bookid; private String title; private int author_id; private int publisher_id; }
Controller
Inside the Package create the class borrowController
@RestController @CrossOrigin @RequestMapping("api/v1/borrow") public class BorrowController { @Autowired private BorrowService borrowService; @PostMapping(path = "/save") public String saveBorroe(@RequestBody BorrowSaveDTO borrowSaveDTO) { String borrowBooks = borrowService.addBorrow(borrowSaveDTO); return "Borrowel Successfully"; } @GetMapping(path = "/getAllBorrow") public List<BorrowDTO> getAllBorrow() { List<BorrowDTO> allborrow = borrowService.getAllBorrow(); return allborrow; } @PutMapping(path = "/update") public String updateBorrow(@RequestBody BorrowUpdateDTO borrowUpdateDTO) { String borrow = borrowService.updateBorrow(borrowUpdateDTO); return "Updatedddd"; } }
Inside the Package create the interface borrowService.java and borrowServiceIMPL.java.
borrowService
public interface BorrowService { String addBorrow(BorrowSaveDTO borrowSaveDTO); List<BorrowDTO> getAllBorrow(); String updateBorrow(BorrowUpdateDTO borrowUpdateDTO); }
borrowServiceIMPL
@Service public class BorrowServiceIMPL implements BorrowService { @Autowired private BookRepo bookRepo; @Autowired private UserRepo userRepo; @Autowired private BorrowRepo borrowRepo; @Override public String addBorrow(BorrowSaveDTO borrowSaveDTO) { Borrow borrow = new Borrow( bookRepo.getById(borrowSaveDTO.getBook_id()), userRepo.getById(borrowSaveDTO.getUser_id()), borrowSaveDTO.getBorrowDate(), borrowSaveDTO.getReturnDate() ); borrowRepo.save(borrow); return null; } @Override public List<BorrowDTO> getAllBorrow() { List<Borrow> getBorrow = borrowRepo.findAll(); List<BorrowDTO> borrowDTOList = new ArrayList<>(); for(Borrow borrow : getBorrow) { BorrowDTO borrowDTO = new BorrowDTO( borrow.getId(), borrow.getBook(), borrow.getUser(), borrow.getBorrowDate(), borrow.getReturnDate() ); borrowDTOList.add(borrowDTO); } return borrowDTOList; } @Override public String updateBorrow(BorrowUpdateDTO borrowUpdateDTO) { try { if(borrowRepo.existsById(borrowUpdateDTO.getId())) { Borrow borrow = borrowRepo.getById(borrowUpdateDTO.getId()); borrow.setBook(bookRepo.getById(borrowUpdateDTO.getBook_id())); borrow.setUser(userRepo.getById(borrowUpdateDTO.getUser_id())); borrow.setBorrowDate(borrowUpdateDTO.getBorrowDate()); borrow.setReturnDate(borrowUpdateDTO.getReturnDate()); borrowRepo.save(borrow); return "Borrow updated successfully."; } else { System.out.println("Borrow ID Not Found"); } } catch (Exception ex) { System.out.println(ex); } return null; }
borrowRepo
public interface BorrowRepo extends JpaRepository<Borrow,Integer > { }