In this Article explains how to create the login and registration using Spring Boot and Vue js and how to set the hash password for the Security .Spring boot as backend front end Vue js.
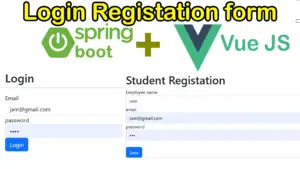
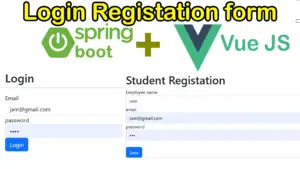
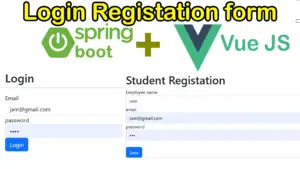
Lets follow the following step
First you have add the following dependencies while you configuring the spring initializr
- spring jpa
- spring web
- spring security
- mysql
Open the project on the pom.xml
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>com.mysql</groupId> <artifactId>mysql-connector-j</artifactId> <scope>runtime</scope> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-security --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> <version>2.7.8</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency>
you see the dependencies what you added.
Create the Mysql Database and Configure the database connection in the application.properties. this place where connect to Spring boot to Mysql.
application.properties
spring.application.name=Registation server.port=8085 spring.jpa.hibernate.ddl-auto=create spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3306/dbkms?createDatabaseIfNotExist=true spring.datasource.username=root spring.datasource.password=root123 #jpa vendor adapter configuration spring.jpa.database-platform=org.hibernate.dialect.MySQL57Dialect spring.jpa.generate-ddl=true spring.jpa.show-sql=true
Create the Entity Employee for represent the employee data in the database.
Employee
package com.example.Registation.Entity; import javax.persistence.*; @Entity @Table(name="employee") public class Employee { @Id @Column(name="employee_id", length = 45) @GeneratedValue(strategy = GenerationType.AUTO) private int employeeid; @Column(name="employee_name", length = 255) private String employeename; @Column(name="email", length = 255) private String email; @Column(name="password", length = 255) private String password; public Employee() { } public Employee(int employeeid, String employeename, String email, String password) { this.employeeid = employeeid; this.employeename = employeename; this.email = email; this.password = password; } //create getters and setters
EmployeeDTO
public class EmployeeDTO { private int employeeid; private String employeename; private String email; private String password; public EmployeeDTO() { } public EmployeeDTO(int employeeid, String employeename, String email, String password) { this.employeeid = employeeid; this.employeename = employeename; this.email = email; this.password = password; } } //create getters and setters
LoginDTO
public class LoginDTO
{
private String email;
private String password;
public LoginDTO() {
}
public LoginDTO(String email, String password) {
this.email = email;
this.password = password;
} //create getters and setters
Create a EmployeeRepository interface which extends JpaRepository
EmployeeRepo
package com.example.Registation.Repo;
import com.example.Registation.Entity.Employee;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.config.EnableJpaRepositories;
import org.springframework.stereotype.Repository;
import java.util.Optional;
@EnableJpaRepositories
@Repository
public interface EmployeeRepo extends JpaRepository<Employee,Integer>
{
Optional<Employee> findOneByEmailAndPassword(String email, String password);
Employee findByEmail(String email);
}
Create the Employee Service
EmployeeService
package com.example.Registation.Service;
import com.example.Registation.Dto.EmployeeDTO;
import com.example.Registation.Dto.LoginDTO;
import com.example.Registation.payload.response.LoginMesage;
public interface EmployeeService {
String addEmployee(EmployeeDTO employeeDTO);
LoginMesage loginEmployee(LoginDTO loginDTO);
}
Create the EmployeeImpl which implements from EmployeeService
EmployeeImpl
package com.example.Registation.Service.impl;
import com.example.Registation.Dto.EmployeeDTO;
import com.example.Registation.Dto.LoginDTO;
import com.example.Registation.Entity.Employee;
import com.example.Registation.Repo.EmployeeRepo;
import com.example.Registation.Service.EmployeeService;
import com.example.Registation.payload.response.LoginMesage;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.Optional;
@Service
public class EmployeeIMPL implements EmployeeService {
@Autowired
private EmployeeRepo employeeRepo;
@Autowired
private PasswordEncoder passwordEncoder;
@Override
public String addEmployee(EmployeeDTO employeeDTO) {
Employee employee = new Employee(
employeeDTO.getEmployeeid(),
employeeDTO.getEmployeename(),
employeeDTO.getEmail(),
this.passwordEncoder.encode(employeeDTO.getPassword())
);
employeeRepo.save(employee);
return employee.getEmployeename();
}
EmployeeDTO employeeDTO;
@Override
public LoginMesage loginEmployee(LoginDTO loginDTO) {
String msg = "";
Employee employee1 = employeeRepo.findByEmail(loginDTO.getEmail());
if (employee1 != null) {
String password = loginDTO.getPassword();
String encodedPassword = employee1.getPassword();
Boolean isPwdRight = passwordEncoder.matches(password, encodedPassword);
if (isPwdRight) {
Optional<Employee> employee = employeeRepo.findOneByEmailAndPassword(loginDTO.getEmail(), encodedPassword);
if (employee.isPresent()) {
return new LoginMesage("Login Success", true);
} else {
return new LoginMesage("Login Failed", false);
}
} else {
return new LoginMesage("password Not Match", false);
}
}else {
return new LoginMesage("Email not exits", false);
}
}
}
Create a EmployeeController to manage the Restful api requests related to employee login and registration.
package com.example.Registation.Dto;
public class EmployeeDTO {
private int employeeid;
private String employeename;
private String email;
private String password;
public EmployeeDTO() {
}
public EmployeeDTO(int employeeid, String employeename, String email, String password) {
this.employeeid = employeeid;
this.employeename = employeename;
this.email = email;
this.password = password;
}
public int getEmployeeid() {
return employeeid;
}
public void setEmployeeid(int employeeid) {
this.employeeid = employeeid;
}
public String getEmployeename() {
return employeename;
}
public void setEmployeename(String employeename) {
this.employeename = employeename;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Override
public String toString() {
return "EmployeeDTO{" +
"employeeid=" + employeeid +
", employeename='" + employeename + '\'' +
", email='" + email + '\'' +
", password='" + password + '\'' +
'}';
}
}
Security measures need to implemented
Create a file SecurityConfig for managing the Security password
package com.example.Registation.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@Configuration
public class SecurityConfig {
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
Create LoginResponse Page for displaying Messages while testing
LoginResponse
public class LoginMesage {
String message;
Boolean status;
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public Boolean getStatus() {
return status;
}
public void setStatus(Boolean status) {
this.status = status;
}
public LoginMesage(String message, Boolean status) {
this.message = message;
this.status = status;
}
}
After competed the Back End.Let’s start the front-end Vue JS.
Vue.js
Install Vue.js CLI
npm install –g vue–cli
After installed successfully create the vue.js project using following command
vue init webpack frontend
Bootstrap
add the bootstrap css on index.html inside the head tag go to the respective website here
you have to wait until the installation process complete.after install successfully.
Open project into vscode editor.
First Step
Create the component Register.vue.
Register.vue
<template> <div class="card" align="left"> <div class="card-header">Register Form</div> <div class="card-body"> <form @submit.prevent="saveData"> <label>Employee Name</label> <input type="text" v-model="employee.employeename" name="employeename" id="employeename" class ="form-control"/> <label>Email</label> <input type="email" v-model="employee.email" name="email" id="email" class ="form-control"/> <label>Password</label> <input type="password" v-model="employee.password" name="password" id="password" class ="form-control"/> <input type="submit" value="Save" class="btn btn-success"> </form> </div> </div> </template> <script> import Vue from 'vue'; import axios from 'axios'; Vue.use(axios) export default { name: 'Register', data () { return { result: {}, employee:{ employeename: '', email: '', password: '' } } }, created() { }, mounted() { console.log("mounted() called......."); }, methods: { saveData() { axios.post("http://localhost:8085/api/v1/employee/save", this.employee) .then( ({data})=>{ console.log(data); try { alert("Employee Registation Successfully"); } catch(err) { alert("failed"); } } ) } } } </script>
Login.vue
<template>
<div class="row">
<div class="col-sm-4" >
<h2 align="center"> Login</h2>
<form @submit.prevent="LoginData">
<div class="form-group" align="left">
<label>Email</label>
<input type="email" v-model="employee.email" class="form-control" placeholder="Email">
</div>
<div class="form-group" align="left">
<label>Password</label>
<input type="password" v-model="employee.password" class="form-control" placeholder="Password">
</div>
<br/>
<button type="submit" class="btn btn-primary">Login</button>
</form>
</div>
</div>
</template>
<script>
import Vue from 'vue';
import axios from 'axios';
Vue.use(axios)
export default {
name: 'Registation',
data () {
return {
result: {},
employee:{
email: '',
password: ''
}
}
},
created() {
},
mounted() {
console.log("mounted() called.......");
},
methods: {
LoginData()
{
axios.post("http://localhost:8085/api/v1/employee/login", this.employee)
.then(
({data})=>{
console.log(data);
try {
if (data.message === "Email not exits")
{
alert("Email not exits");
}
else if(data.message == "Login Success")
{
this.$router.push({ name: 'Home' })
}
else
{
alert("Incorrect Email and Password not match");
}
} catch (err) {
alert("Error, please try again");
}
}
)
}
}
}
</script>
HelloWorld.vue
<template>
<div>
<h1>Welcome to Home Page</h1>
</div>
</template>
<script>
export default {
name: 'Home',
data () {
return {
msg: 'Welcome to Your Vue.js App'
}
}
}
</script>
Routes
all routes manage in vuejs by inside the routes folder index.js
import Vue from 'vue'
import Router from 'vue-router'
import Register from '@/components/Register'
import Login from '@/components/Login'
import Home from '@/components/Home'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/register',
name: 'Register',
component: Register
},
{
path: '/',
name: 'Login',
component: Login
},
{
path: '/home',
name: 'Home',
component: Home
}
]
})
i have attached the video link below. which will do this tutorials step by step.