Introduction
In this section, we will guide you step by step in the development of an image upload registration system in Java using MySQL and JDBC. In the application, users register by providing their information and an image (profile photo), which will be stored in the database. If you want a live Java project with source code, you are welcome here.
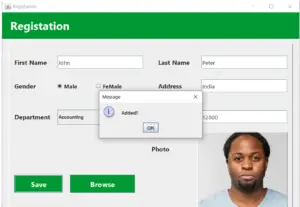
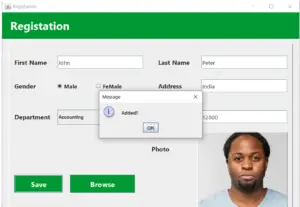
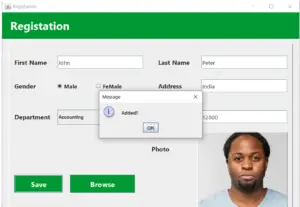
Project Features
- User Registration: Users can input their firstname,lastname,address,department,photo,salary.
- JDBC Connectivity: Get secure connection from Java to MySQL.
- Simple & Secure: Adheres to the best practices for database interaction.
Here is Code
Establish the database Connection
public navigationform() { initComponents(); connect(); } private Connection con; private PreparedStatement pst; private ResultSet rs; private byte[] userimage; private String realativePath; public void connect(){ try { String driver = "com.mysql.cj.jdbc.Driver"; String url = "jdbc:mysql://localhost:3306/navication"; // Correct JDBC URL String un = "root"; String pw = ""; Class.forName(driver); con = DriverManager.getConnection(url, un, pw); System.out.println("Database Connected Successfully!"); } catch (ClassNotFoundException ex) { Logger.getLogger(navigationform.class.getName()).log(Level.SEVERE, null, ex); } catch (SQLException ex) { Logger.getLogger(navigationform.class.getName()).log(Level.SEVERE, null, ex); } }
browse button
JFileChooser picchoose = new JFileChooser(); picchoose.showOpenDialog(null); File pic = picchoose.getSelectedFile(); String path = pic.getAbsolutePath(); BufferedImage img; try { img = ImageIO.read(picchoose.getSelectedFile()); ImageIcon imageic = new ImageIcon(new ImageIcon(img).getImage().getScaledInstance(250,250,Image.SCALE_DEFAULT)); lblPhoto.setIcon(imageic); File imageDir = new File ("src/images"); if(!imageDir.exists()){ imageDir.mkdirs(); } File imageFile = new File (imageDir, pic.getName()); Files.copy(pic.toPath() , imageFile.toPath() , StandardCopyOption.REPLACE_EXISTING); realativePath = "src/images/" + pic.getName(); FileInputStream fis = new FileInputStream(imageFile); ByteArrayOutputStream bos = new ByteArrayOutputStream(); byte[] buff = new byte[1024]; for(int readNum; (readNum=fis.read(buff)) !=-1;) { bos.write(buff,0,readNum); } userimage = bos.toByteArray(); } catch (IOException ex) { }
Add Function
public void add(){ try { String gender; String fname = txtFname.getText(); String lname = txtLname.getText(); String address = txtAddress.getText(); if(radioMale.isSelected()) { gender = radioMale.getText(); } else{ gender = radioFemale.getText(); } String dep = (String) ComboDepar.getSelectedItem(); String path = lblPhoto.getText(); String salary = txtSalary.getText(); pst = con.prepareStatement("insert into navi (firstname,lastname,address,gender,department,path,basicsalary) values(?,?,?,?,?,?,?)"); pst.setString(1, fname); pst.setString(2, lname); pst.setString(3, address); pst.setString(4, gender); pst.setString(5, dep); pst.setString(6, realativePath); pst.setString(7, salary); pst.executeUpdate(); JOptionPane.showMessageDialog(this,"Added!!"); } catch (SQLException ex) { Logger.getLogger(navigationform.class.getName()).log(Level.SEVERE, null, ex); }
Call inside the Add Button
add();
Find the Records
public void find(){ try { int id = Integer.parseInt(txtId.getText()); pst = con.prepareStatement("select firstname,lastname,address,gender,department,path,basicsalary from navi where Id = ?" ); pst.setInt(1, id); rs = pst.executeQuery(); if(rs.next() == true) { String fname = rs.getString("firstname"); String address = rs.getString("address"); String dep = rs.getString("department"); String basic = rs.getString("basicsalary"); String lname = rs.getString("lastname"); String gender = rs.getString("gender"); String imagepath = rs.getString("path"); if ("Male".equalsIgnoreCase(gender)) { radioMale.setSelected(true); } else { radioFemale.setSelected(true); } System.out.println("Image path retrieved: " + imagepath); File imageFile = new File(imagepath); if(imageFile.exists()) { BufferedImage img = ImageIO.read(imageFile); ImageIcon imageIcon = new ImageIcon (new ImageIcon(img).getImage().getScaledInstance(250, 250, Image.SCALE_DEFAULT)); lblPhoto.setIcon(imageIcon); System.out.println("Image displayed successfully."); } else { System.out.println("Image file does not exist at path: " + imagepath); } txtFname.setText(fname); txtLname.setText(lname); txtAddress.setText(address); txtSalary.setText(basic); ComboDepar.setSelectedItem(dep); } } catch (SQLException ex) { Logger.getLogger(Finding.class.getName()).log(Level.SEVERE, null, ex); } catch (IOException ex) { Logger.getLogger(Finding.class.getName()).log(Level.SEVERE, null, ex); }